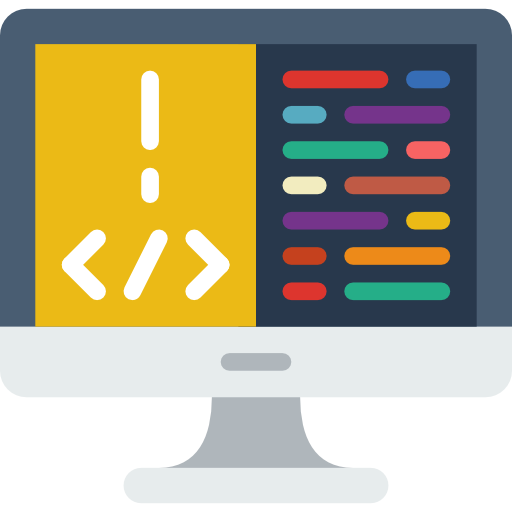
Python Interview
1 of 30 Completed
Introduction
numpy
scipy
matplotlib
pandas
sklearn
Over 100 Dollars
Good Grades and Favorite Colors
Generate Normal Distribution
Random Seed Function
Complete Addresses
numpy
Due to Python’s list class being used as its main iterable, Python does not allow much traditional functionality given by the equivalent iterables in other languages. For example, there is no way to initialize a specific list of lists with a certain number of rows and columns.
numpy adds this functionality via a class known as a numpy array. For example, the following code initalizes a numpy array of zeros with 4 rows and 3 columns:
import numpy as np
A = np.zeros((4,3)) A
numpy was created as a linear algebra liberary, so it provides for standard linear algebra operations like matrix multiplications, inversion, and solving linear systems. Try running the following in a Jupyter Notebook:
A = np.array([[1,2,2],[4,4,4],[7,8,9]]) B = np.array([[7,8,9],[4,4,4],[1,2,3]]) print(‘A:’) print(A) print(‘B:’) print(B) print()
Matrix multiplication
print(‘AB:’) print(AB) print()
Inverse
print(‘A^{-1}:’) print(np.linalg.inv(A)) print()
Solve Linear equation
y = np.array([10,20,30]) print(‘Solution to Ax=[10,20,30]’) print(np.linalg.solve(A,y))
Additionally, numpy has an alternative to the None class known as nan that is useful for describing missing data in many datasets. numpy also provides functionality for infinite via its inf class, which is useful for some computational problems.
3%
CompletedYou have 29 sections remaining on this learning path.
Advance your learning journey! Go Premium and unlock 40+ hours of specialized content.